1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| #include <iostream> #include <functional> using namespace std; class A { public: A() :m_a(0){} ~A(){} virtual void SetA(const int& a){ cout << "A:" << this << endl; m_a = a; } int GetA()const { return m_a; } protected: int m_a; }; class B: public A { public: B():A(){;} ~B(){;} virtual void SetA(const int& a){ cout << "B:" << this << endl; m_a = a; } private: }; int main(void) { A a; cout << "A:" << &a << endl; function<void(const int&)> func1 = std::bind(&A::SetA, a, std::placeholders::_1); func1(1); cout << a.GetA() << endl; function<void(const int&)> func2 = std::bind(&A::SetA, &a, std::placeholders::_1); func2(2); cout << a.GetA() << endl; cout << "---------" << endl; A* pa = new B(); cout << "B:" << pa << endl; function<void(const int&)> func3 = std::bind(&A::SetA, pa, std::placeholders::_1); func3(3); cout << pa->GetA() << endl; function<void(const int&)> func4 = std::bind(&A::SetA, *pa, std::placeholders::_1); func4(4); cout << pa->GetA() << endl; delete pa; system("pause"); return 0; }
|
输出是:
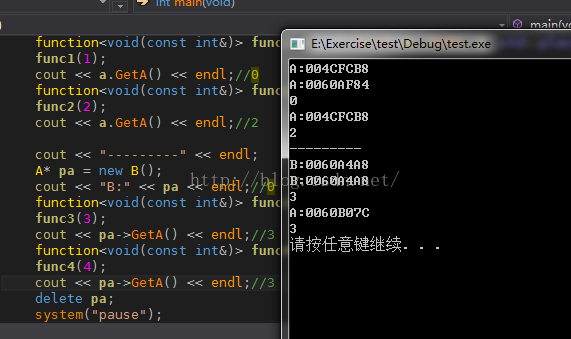
由输出可以看出:
1、func1调用时产生了一个临时对象,然后调用临时对象的SetA;
2、func2调用的是a.SetA,改变了对象a中m_a的值;
3、func3调用的是pa->SetA,输出B:0060A4A8,调用的时B的SetA改变了pa->m_a;
4、func4调用时产生了一个临时对象,然后调用临时对象的A::SetA;
结论:std::bind中第二个参数应该是对象的指针,且std::bind支持虚函数。